If you’ve ever used an API without documentation, you know the frustrating experience of blundering through endless trial and error. You’d keep trying to understand what endpoints do and why your request keeps generating error messages. This is why API documentation is important. So, what are API docs?
Good API documentation acts as a roadmap for developers. It lists the methods, endpoints, error messages, and all requirements for smooth usage. Besides conserving time, good API documentation dramatically improves the user experience, helping developers to integrate an API as seamlessly as possible.
This guide covers everything you need to know about API documentation. By the end, you’ll know how to write API documentation and the different components to include. We’ll also cover some of the most effective API documentation tools and best practices for creating your API documentation.
What is API Documentation
API documentation is essentially an API’s user manual. It tells developers how to use an API, what it can do, and how to interact with its endpoints. Besides being a technical document API documentation also serves as a communication tool for bridging the gap between an API’s functionality and its audience.
At its core, API documentation should answer the following questions:
What does the API do?
It should explain the general purpose and value of the API.
How does it work?
It must provide the technical details, such as how to authenticate, make requests, and handle answers.
What are the edge cases or potential errors?
It should provide guidance on error codes and troubleshooting.
It doesn’t matter what type of API it is, these fundamental building blocks allow developers to build, debug, and maintain their integrations efficiently.
Key Components of Effective API Documentation
What should you add to your API documentation? Here’s a breakdown to help you:
1. Overview and Purpose of the API
The Overview and Purpose section should establish the context for the API and set expectations for what it does. It gives developers a quick insight into what the API does and what types of problems it can solve.
What to Include:
- General Description: A brief description of the purpose of your API, for example, “Our API allows online businesses to fully integrate payment processing into their business”.
- Use cases: Clearly explain how someone would use it — eg “This program allows developers to automate financial trades, manage client and customer information, or generate invoices.”
- User: Specify who will benefit most, for example, web developers, mobile app builders, backend engineers, etc.
Example:
2. Endpoints
Endpoints tell the user the route that will give them access to the API’s functionality. An endpoint is either a resource (ie, a specific source of data) or an action (ie, a request that can be made). They should be completely understandable from their description.
What to Include:
- Endpoint URL: Clearly specify the URL path for each endpoint.
- HTTP methods: Indicate which methods are supported (GET, POST, PUT, DELETE, etc.).
- Endpoint purpose: Give a short description of the endpoint function (eg. get user data, or create a new order).
Example:
GET /users/{user_id}
– This endpoint retrieves details of a specific user by their unique user ID.
3. Methods/Operations
API methods, often called HTTP methods, or operations, are the actions you can perform on the resource. Each method reflects a specific interaction type, such as getting, saving, updating, or deleting data.
What to Include:
- Supported methods: List out all the supported HTTP methods (e.g., GET, POST, PUT, DELETE).
- Purpose of each method: Explain what each method does when applied to an endpoint.
- Method-specific details: Explain any nuances specific to a method, like how a POST request to create a resource may differ from a PUT request to update one.
Example:
For the /users
resource:
- GET: Retrieve a list of users or a single user by ID.
- POST: Create a new user.
- PUT: Update an existing user’s information.
- DELETE: Remove a user from the database.
4. Request and Response Formats
The structure of requests and responses is the key to making successful API calls. This section is especially important for making developers aware of what to send as part of a request, as well as the content they should be able to expect in the form of a response.
What to Include:
- Request format: Describe what developers should send in their requests (e.g., JSON, XML).
- Required/optional fields: Specify what fields need to be completed and which are optional.
- Response structure: Show what the API will return after a request, such as required fields and data types.
Example Request:
POST /users
Content-Type: application/json
{
"name": "Jane Doe",
"email": "[email protected]"
}
Example Response:
{
"id": 2,
"name": "Jane Doe",
"email": "[email protected]"
}
5. Parameters and Headers
Parameters and headers are crucial parts of any API request, and documenting their usage correctly will help developers know exactly what they should send for a particular request to work. In this section, you’ll explain which parameters are required to be sent or not, and how headers should be formatted.
What to Include:
- Query Parameters: Define optional or required parameters sent in the URL.
- Path Parameters: These are typically required, such as
user_id
inGET /users/{user_id}
. - Request Headers: Explain necessary headers like
Content-Type
andAuthorization
.
Example:
For the endpoint GET /users/{user_id}
, the user_id
of the user should be passed as a path parameter, and the request must have an Authorization
header with a valid API key.
6. Authentication
Many APIs require authentication to ensure only qualified users can interact with certain resources. This section should explain which authentication mechanisms are supported by your API and show how to employ them.
What to Include:
- Authentication methods: Explain whether the API uses API keys, OAuth, or another method.
- Example usage: Enter sample requests where you illustrate how to include authorization information in headers or in parameters.
- Token expiration: If applicable, clarify how long tokens last and how to refresh them.
Example:
For APIs using Bearer tokens:
GET /users/me
Authorization: Bearer <your_access_token>
7. Error Codes and Messages
Good error handling helps the developer to diagnose and fix issues quickly when integrating. Listing error codes and messages let you know what went wrong when requests fail.
What to Include:
- Common error codes: List typical HTTP status codes (e.g., 400, 401, 404, 500) with explanations.
- Error messages: Include human-readable descriptions for each error to help developers understand the issue.
- Troubleshooting tips: Optionally, provide hints on how to resolve specific errors.
Example:
404 Not Found
: The requested resource doesn’t exist.401 Unauthorized
: Authentication failed or missing.
8. Rate Limits
Protection against abuse is a key reason why APIs are often coupled to a set of rules, known as rate limits. These limits allow users to send only a certain number of API requests over a specific period of time. Documenting rate limits can help developers avoid hitting them, alerting them to situations when they’re required to wait before sending more requests, and guiding their use of quotas when they have them.
What to Include:
- Rate limits: Specify how many requests are allowed per minute, hour, or day.
- Response to exceeding limits: Explain what happens when the limit is exceeded (e.g., HTTP 429 Too Many Requests).
- Best practices: Optionally, provide further tips that help address rate limits, like how to implement retries or request pacing.
Example:
Rate Limit: 100 requests per minute
Response to exceeding rate limit:
{
"error": "Rate limit exceeded",
"code": 429
}
9. Sample Code Snippets
This section provides the developers with pre-coded examples to help with integrations. It includes code snippets in different common languages (like Python, JavaScript, or PHP), which can cut down integration time and frustration.
What to Include:
- Common languages: Provide examples in widely-used programming languages, like Python, JavaScript, or Node.js.
- Basic operations: Describe what is required to achieve certain desirable outcomes.
- Practical use cases: Go beyond the usual “Hello World” examples; demo some real-world scenarios that the API might be intended to serve.
Example:
Here’s how to retrieve user information using Python:
import requests url = 'https://api.example.com/users/12345' headers = {'Authorization': 'Bearer <your_api_key>'} response = requests.get(URL, headers=headers) print(response.json())
10. Contact Information for Support and Feedback
This is often an overlooked yet critical part of any good API documentation. Developers can hit snags or might want to make more inquiries. Clear contact points ensure that they know where to go when they need help.
What to Include:
- Email address: Provide an easily accessible support email.
- Support forums or community links: If your organization or startup has a developer forum, add the link here.
- Documentation feedback: Optionally, include a method for developers to suggest improvements or report issues.
Evolution of Documentation Throughout the API Lifecycle
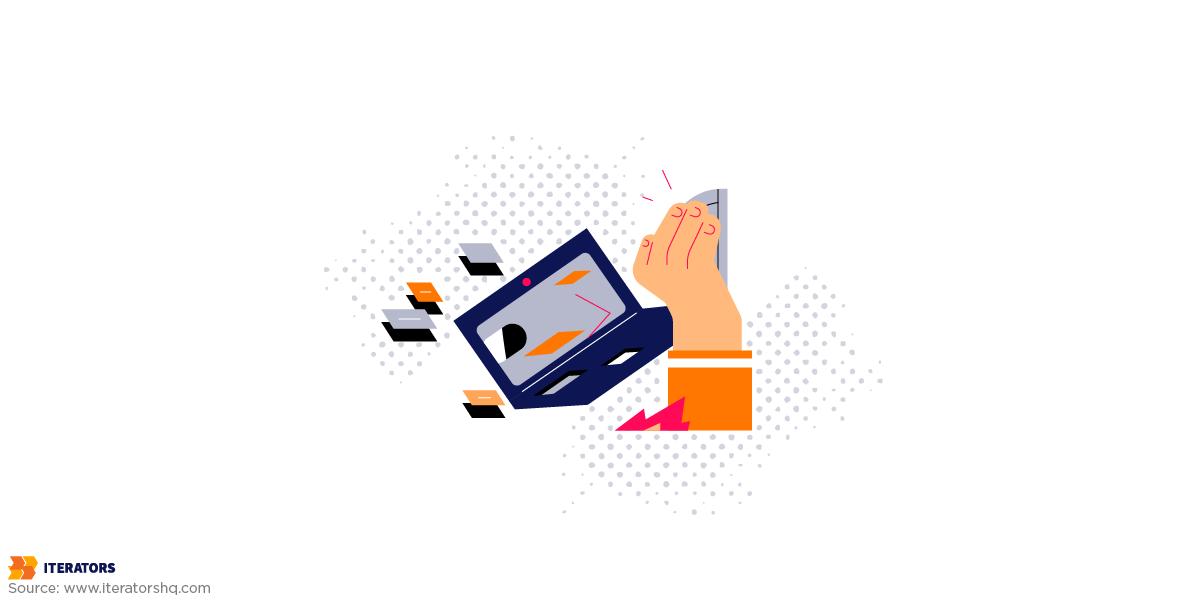
When implemented effectively, API documentation does more than just act as a supplementary guide. It becomes an ongoing source of information that spans the lifecycle of an API – from design to development to support. Only through complete and accurate documentation is it possible to keep an API comprehensible and useful as it evolves with each new feature, update, or bug fix.
Maintaining this evolutionary process involves organization and following best practices so that documentation stays as synced as possible with an API’s iterative roadmap.
How API Documentation Evolves Through Design, Development, and Maintenance
During the API design phase, documentation often starts with conceptual overviews, explanations of the endpoints available, and the specific data models provided. This documented information is used primarily as a form of API communication at the design stage. It outlines the proposed structure for the API along with a description of how it’s supposed to function. This might include mock responses or other forms of sample data to help stakeholders and developers envision what the API will do.
Documentation built in the development phase can include anything from an actual request and response example to detailed explanations of how errors should be handled. It also specifies exactly how the endpoints should look and which parameters they should accept. As developers code an API, they often create documentation directly from their implementation using tools such as Swagger or RAML. This approach ensures consistency between the documented API and what developers code.
After the API has been created and launched, maintenance extends well beyond mere code updates. The API documentation must stay in sync with these evolutions, which might include new features or what’s known as breaking changes to the API. This is important for both the internal development team and for third-party developers who continue to work on integrations with the ever-evolving internal API. If the actual API changes and the documentation does not change, it is likely that clients will continue to write code to an outdated version of the API, risking miscommunication and integration errors.
Strategies for Keeping Documentation Up-to-Date
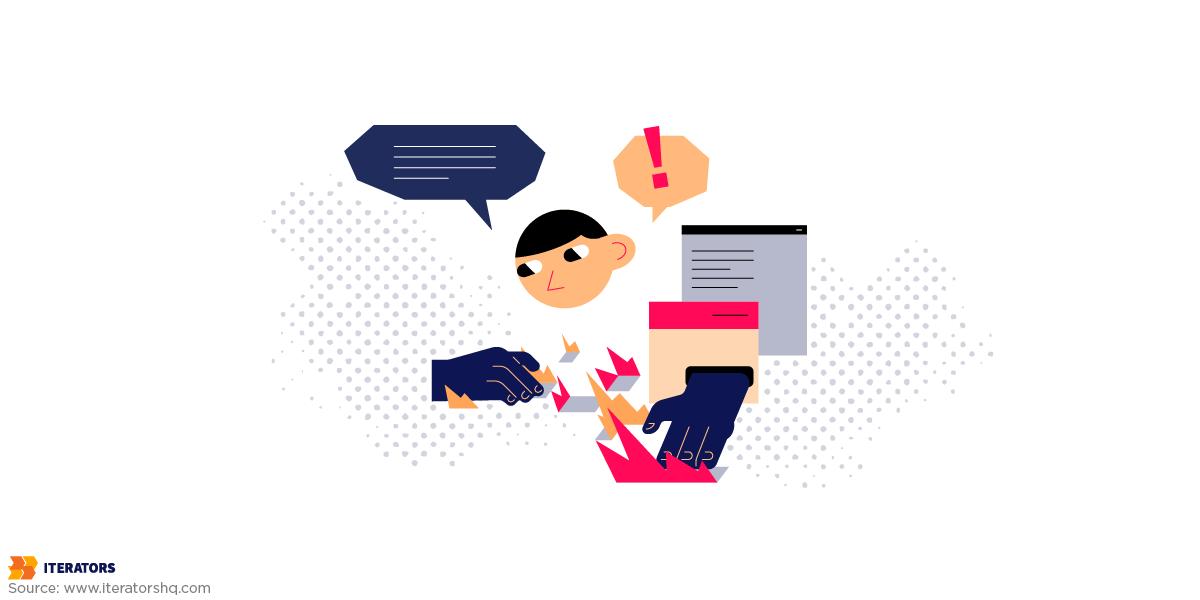
Ensuring that documentation evolves along with the API requires specific strategies. Some of the most effective approaches include:
1. Automated documentation tools
Tools such as SwaggerHub, Postman, and Stoplight automatically generate and update API documentation from the codebase itself. This removes much of the error-prone human touch, as well as the risk of miscommunicating the API’s function.
2. Versioning
As APIs age, they require versioning not only of the API design but of its documentation as well. It’s important to highlight changes between versions so developers can know what has changed, and how they can adopt newer versions without breaking their existing integrations. A change log, alongside a deprecation notice, should thus become an integral part of the API documentation.
3. Documentation reviews
Checking the documentation at regular intervals (for example, every time you make a major release) is essential to keep it current. Designate a development time early on in each cycle to clean up the documentation and track its progress against pending milestones. Also, pay careful attention to the documentation during a release as it may help you catch documentation issues early.
4. User Feedback
Listening to feedback from API users can be a good way to identify areas you didn’t cover in the documentation. If you regularly receive reports of issues or confusion from developers using certain endpoints or features, that may be a sign that the documentation there could be clearer or more detailed. Feedback can be a helpful way to integrate users into the process of updating the documentation.
How Protocols and Formats Influence Documentation Standards
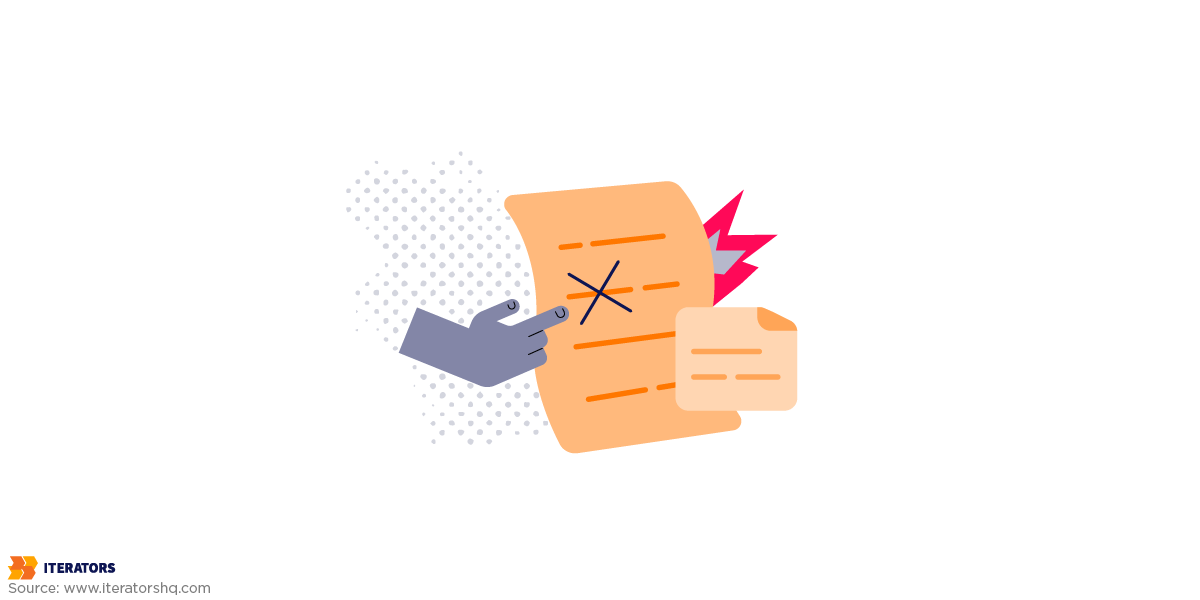
The protocols and formats used in an API help in shaping how the documentation is structured and presented. Here’s how various protocols and formats influence API documentation standards:
1. REST (Representational State Transfer)
REST APIs transmit data using JSON or XML. Tools like OpenAPI (previously Swagger) are popular for REST API documentation. This platform provides a way to standardize how the endpoints, parameters, and data models are documented.
The OpenAPI specification is designed to allow developers to generate documents automatically. The Swagger UI tool includes the option to test API calls right from the documentation. Developers can execute simple requests without having to switch to a code editor and write the same HTTP requests manually. Moreover, in Swagger UI, each API call includes a visualization of the path or parameters being modified.
Structure: REST APIs are arranged around resources and HTTP methods (GET, POST, PUT, DELETE). The docs should clearly indicate which is which, the HTTP method associated with a given resource, and the expected format of the request/response.
2. SOAP (Simple Object Access Protocol)
SOAP APIs use XML for messaging, and the spec needs to clearly define the schema required for the SOAP messages, including the envelope, the header, and the body.
WSDL (Web Services Description Language) and XSD (XML Schema Definition) are often used to document this.
Structure: The documentation must describe exactly which operations are supported by SOAP, the XML schema of the data exchange, and the fine details of how the SOAP envelopes and answers are formed.
3. GraphQL
A GraphQL API allows a client to specify exactly what data they’re looking for, in a human-sounding and flexible query syntax. The output is typically in JSON format. Since it’s typically easier for a developer to understand GraphQL as a query language, schemas, and requests are written in this format.
Now, imagine your GraphQL API hits the market and people want to know how to write queries and mutations for it. You’ll need to document your API to explain what fields and types are available. Tools like GraphiQL and GraphQL Playground provide an interactive environment where you can type queries or mutations, and see the anticipated response to that input. They allow developers to test queries and explore the schema in real-time.
Structure: Because of GraphQL’s lack of definitive endpoints, there is no documentation on specific resources; instead there is documentation on the schema, which describes the available types, fields, queries, and mutations. Developers then need to be shown how to construct queries so as to receive back only the data that they need.
4. gRPC (Google Remote Procedure Call)
gRPC uses Protocol Buffers (Protobuf) for serializing structured data, which means you need to document the Protobuf message structures and the services you’re providing in a different way than REST APIs.
Because gRPC is so dependent on code generation, its documentation often includes .proto
files that define the message formats and service methods. These files are used to generate client libraries for each developer to work with the API.
Structure: gRPC documentation typically includes the definitions of the service, methods available on that service, and the messages related to those methods. The definition of the request/response object and the Protobuf protocol must be crystal clear in the documentation and should mention how to encode the data using Protobuf.
5. Asynchronous APIs (WebSockets, AsyncAPI)
Asynchronous APIs such as those using WebSockets or a message broker frequently use event-driven communication. So, documentation must concentrate on the structure of messages being sent and received as well as being able to handle real-time events.
AsyncAPI is a popular specification for documenting asynchronous APIs. It defines what channels are used, what the message format is, and what type of response or acknowledgment is expected.
Structure: For each asynchronous API, the documentation should describe how clients can subscribe to events, how each type of event message should be formatted, and whether there are expected responses (for success) or indicators of failure to the messages.
How API Documentation Tools Streamline Development Workflows
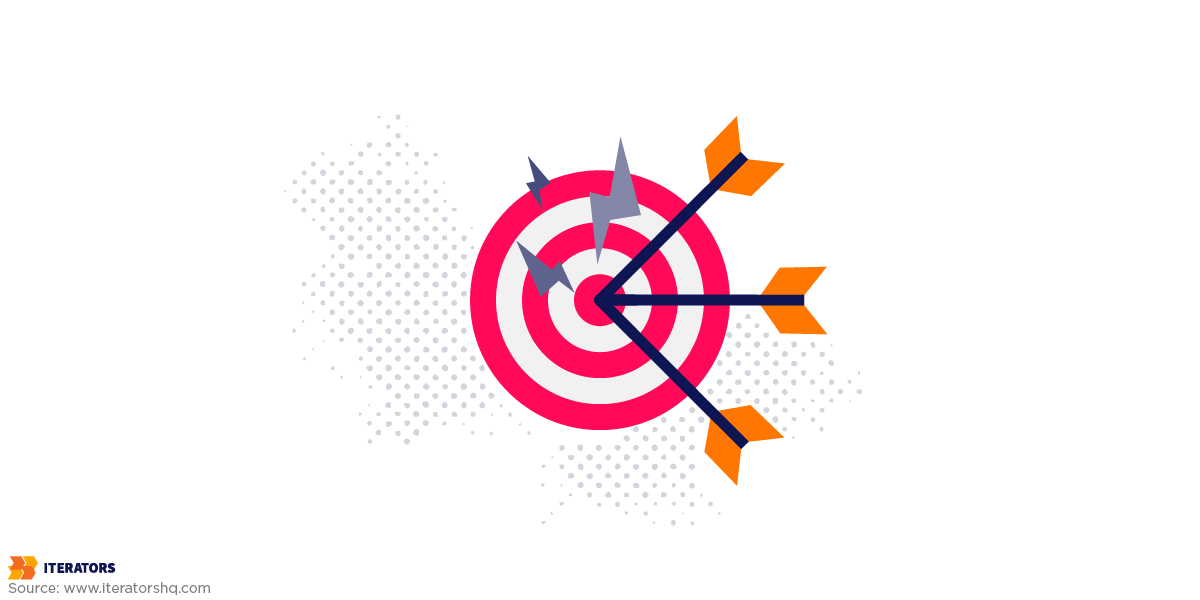
API Documentation tools not only help in crafting rich and interactive documentation but also promote workflow efficiency in the following ways:
1. Real-time API Testing and Debugging
Tools like Postman, Swagger UI, and Insomnia allow developers to send a live API request directly from the documentation interface. This means that when developers see an endpoint they want to test, they can directly and instantly validate the response and catch broken endpoints (or, something else) without ever leaving the tool.
With instant feedback on request-response behavior, developers could iterate on the code much more quickly, catching grammar mistakes at the beginning of the software development pipeline — errors that would otherwise be more costly down the line.
2. Collaboration Across Teams
Some API documentation tools allow teams to save and share collections of API requests. This way, every member of the development team starts from the same API definitions and tests. Some tools also have built-in versioning which makes it easy to track the timeline of API changes. This comes in handy when teams are rapidly iterating over their API.
Platforms such as Prism can render simulated servers offline from API documentation so that a frontend and backend team can work in parallel, greatly reducing downtime. Frontend and backend teams can now operate side by side and still move forward autonomously on development projects without having to wait for the other to complete their set of tasks.
3. Automated Code and Client SDK Generation
You can derive client SDKs and server stubs in multiple programming languages from tools such as Swagger UI and OpenAPI. This feature further reduces the amount of boilerplate code that the developer needs to write to implement an API. This ensures consistency between the API description and the implementation.
Moreover, automatically produced code is less likely to carry human errors. This smoothens project management as client-side and server-side codebases become more tightly aligned. Well-documented APIs can also prevent business logic flaws and their impact which might include the introduction of critical errors into software development.
4. Centralized Documentation for Easy Access
By keeping all API documentation in one place, API tools provide developers with a single source of truth about an API. This reduces confusion and enables developers to locate the information they need easily.
Most documentation tools now feature intuitive UIs with search functionality and endpoint filtering. These features make it possible to start exploring the developer portal and making API calls, without having to trawl through a pile of documentation or put much effort into finding what they need.
5. Simplified Testing and Validation
Some API tools like Postman and Insomnia allow you to write API test scripts and run those test scripts as part of a continuous integration (CI) pipeline. So, you’ll know your APIs still work every time you push any new code.
These tools also come with utilities for checking whether API returns are valid (eg, OpenAPI, JSON Schema validator). This makes sure that the integration continues to receive the exact same responses when it’s running, and you won’t have to deal with inconsistencies later on.
6. Reducing Onboarding Time for New Developers
API documentation tools with interactive features (like try-it-now buttons or query builders) help new developers understand the API faster. Instead of reading through dense text, they can experiment with live endpoints and learn by doing.
With features like sample code snippets and pre-configured requests, you can onboard developers effectively since they can start right away without having to write requests from scratch.
Popular Tools for Generating and Interacting with API Documentation
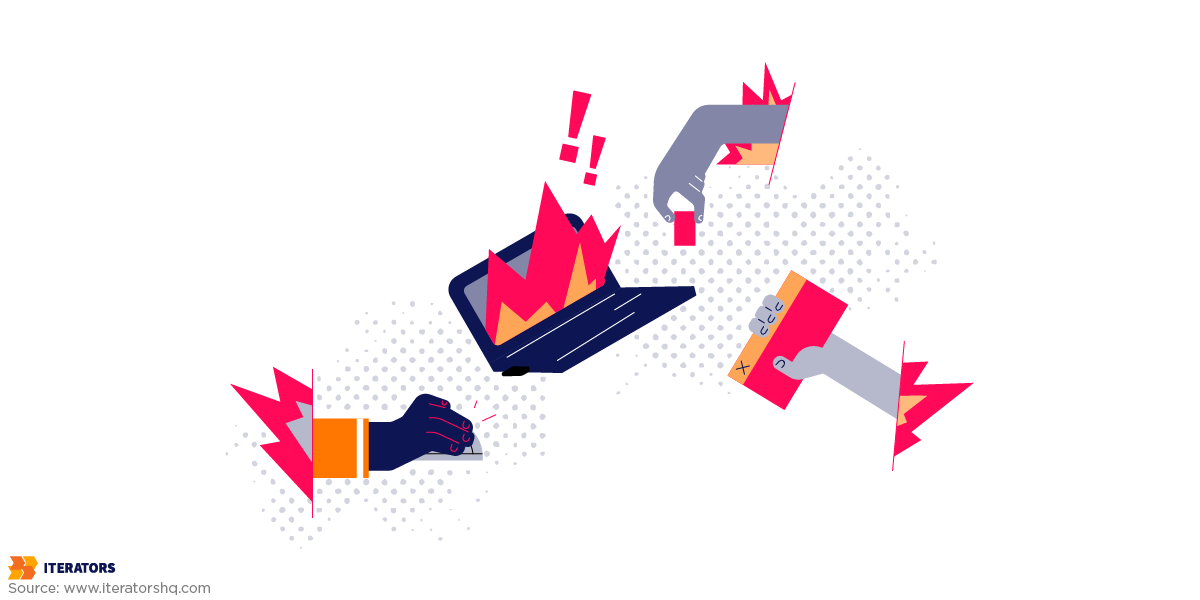
Here are some popular tools that can improve your API documentation experience:
1. Postman
Most developers will consider Postman to be the best API documentation tool so far. It’s commonly used to send HTTP requests to endpoints, inspect the responses, and automatically generate collections of mock API calls used for testing or to be published as documentation.
Features:
- Interactive API testing: Postman makes it easy to test APIs without writing any code.
- Auto-generated documentation: Developers can export API requests and responses into human-readable documentation.
- Collaboration: Teams can share API collections, enabling collaboration across different stages of development.
2. Swagger UI (OpenAPI)
Swagger UI provides a user interface that allows end users and developers to interact with an API based on an OpenAPI specification. The user interface is generated automatically from the OpenAPI specification. Swagger UI presents the available API endpoints and allows the developer or end user to read the request and response formats, populate the request, and send it to the API for execution and response.
Features:
- Live API testing: Users can send requests and view responses directly in the browser.
- Code generation: Swagger Codegen can create client SDKs in multiple languages.
- API exploration: With all the endpoints and paths listed, it’s easy for users/developers to understand how to interact with the API.
3. Insomnia
Insomnia is an open-source API client for REST and GraphQL APIs. It has a straightforward interface that makes it easy to send requests, test APIs, and manage environments.
Features:
- Environment management: Easily manage API requests for different environments (development, staging, production).
- Built-in GraphQL support: Developers can write and test GraphQL queries.
- Plugin support: Users can extend the tool’s functionality with community-developed plugins.
4. GraphQL Playground
GraphQL Playground is built specifically for GraphQL APIs. It’s an excellent sandbox for querying and inspecting your data. GraphQL’s standout features are the interactive schema visualization tool and its query inspector, which is excellent for exploring complex GraphQL schemas and their data types.
Features:
- Interactive querying: Developers can write GraphQL queries and view real-time responses.
- Schema exploration: Visualize the API’s schema and browse available types, fields, and queries.
- Custom headers: Set up custom headers like authentication tokens for API requests.
Key Differences Between Postman, Swagger UI, Insomnia, and GraphQL Playground
Here are the differences between these API documentation tools at a glance:
Feature | Postman | Swagger UI (OpenAPI) | Insomnia | GraphQL Playground |
Primary purpose | API testing, documentation, collaboration | Interactive API documentation from OpenAPI specs | REST/GraphQL API testing and environment management | GraphQL API testing and schema exploration |
Supported protocols | REST, GraphQL, SOAP, gRPC | REST (OpenAPI) | REST, GraphQL | GraphQL |
Auto-generated documentation | Yes (from API requests) | Yes (from OpenAPI spec) | No (manual documentation only) | No (focuses on query testing, not doc generation) |
Code generation | No (available through Newman) | Yes (SDKs via Swagger Codegen) | No | No |
Plugin support | Limited | No | Yes (community plugins) | No |
Key Considerations When Choosing API Documentation Tools
Choosing the right API documentation tool can make the difference between a cumbersome development process and a simplified one.
The right tool supports technical aspects of your API and also improves developer experience and workflow, regardless of the person creating or using your API. Here are the main factors to consider:
1. Simplicity
Ease of use should be a primary consideration. One of the first criteria for any tool for this purpose is that it be usable by more than just API developers. The tool needs to have an intuitive interface so that non-technical users and stakeholders can understand the API, too. The simpler you can make it, the better.
Another aspect of usability is the ease of integration. How well does the tool blend into your existing workflow? Tools that automatically generate documentation from within your codebase (such as Swagger) reduce the amount of manual work it takes to keep documentation up to date.
2. Type of API
Before you pick a documentation tool, you need to figure out what kind of API you’re working with. RESTful APIs are the most common format online, but they’re not the only one. Other protocols include GraphQL, gRPC, and SOAP, and each has slightly different documentation requirements.
- REST APIs: Use SwaggerHub and Postman for RESTful APIs. They provide a rich set of interfaces for OpenAPI specifications and automatically generate detailed interactive documentation for you.
- GraphQL: A developer experience tool such as Apollo Studio or GraphQL Playground can be used to both document your queries, mutations, and subscriptions and allow you to interactively query your API.
- gRPC: APIs built with gRPC most often benefit from tools that can automatically generate documentation in protocol buffer (protobuf) format, such as Buf, or from gRPC-specific plugins for Swagger.
3. Comprehensiveness
It’s good to balance simplicity and complexity in engineering approaches. Even though it’s good to keep things simple, the documentation should also be thorough enough to cover all aspects of the API. What are your needs? Pick the tool that meets your requirements first, and then check off the fancier features. Some of the most critical features are:
- Interactive Documentation
- Version Control
- Code Generation
- Collaboration
Tailoring Documentation for Complex Operations
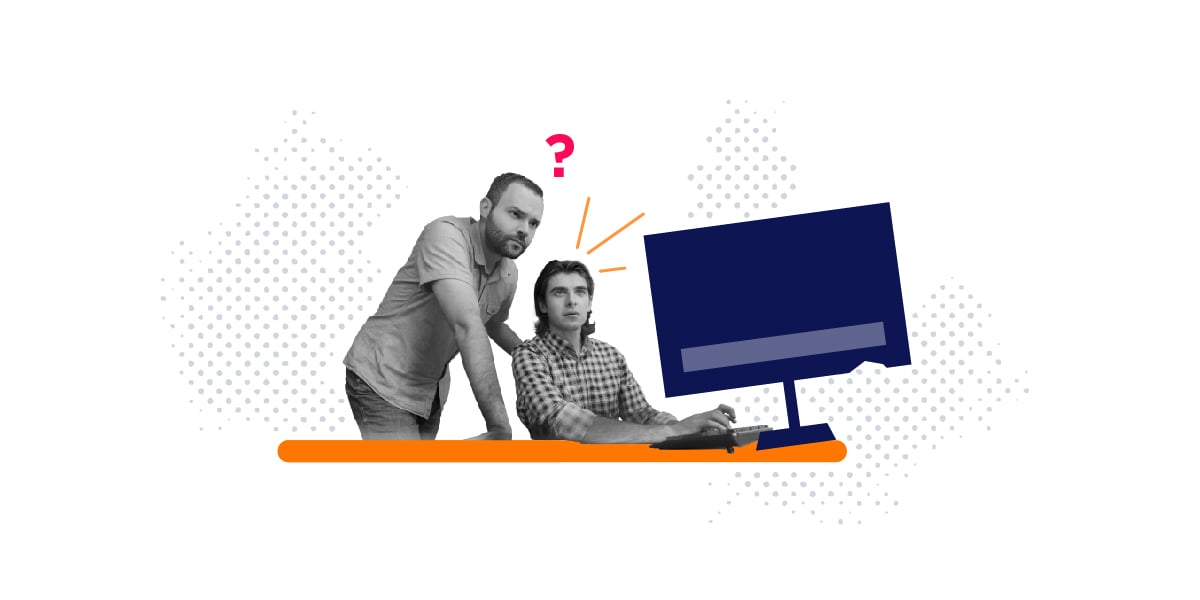
Documenting a simple operation is fairly straightforward. However when creating documentation for complex API operations, there are a few guidelines you must follow to make it simpler for users. These include:
1. Addressing Non-Atomic and Multi-Step Operations
When documenting complex API operations, it’s often necessary to break them into smaller tasks. Non-atomic operations are often built from multiple sequences of API calls that are conditionally dependent on one another, or that need to be called in a specific order. In the case of an order processing API in an e-commerce platform, the flow of events in the platform might look like this:
creating a cart >> adding items >> applying discount codes >> processing payment >> confirming shipment.
With each of these steps, both textual description and samples will be required, capturing:
- Execution sequence: Reorder the steps to reflect the right order in which the operations should be performed.
- Dependencies: Specify the constraints for each step to work (e.g., the cart needs to be created before items can be added).
Sometimes, such operations might be implemented with conditional logic, meaning various branches or responses will kick in depending on the state of resources or the outside world. Mark out these scenarios in your docs so developers can get cues about what to do in each particular case. For example, what happens if the condition isn’t met? Should the operation be retried? Or rolled back? Or have some fallback plan in place?
2. Explain Dependencies and Conditions
Many operations on complex systems are not independent, but tied to dependencies between parts of the system. What this means is that some actions can’t be taken if others haven’t happened first. For example, if an order in a workflow can only be edited if it has not been shipped, developers who write the code for editing need to know what conditions must be held to move forward. These dependencies should be clearly outlined:
- Preconditions: Specify any conditions that must be met before a certain API action can occur, such as user permissions, the state of other resources, or external data.
- Error Handling: Provide details of what will occur when the preconditions are not met, and guidance and/or suggestions for how the consumer can recover or proceed in those cases. This might include an explanation of error codes, status responses, and suggested corrective actions.
Error handling and recovery options become especially important for multi-step procedures. The developer needs to know what action to take if an operation is aborted halfway through, and how they can call the operation back or roll forward or back to a prior state.
3. Provide Detailed Examples and Use Cases
A detailed API documentation example is useful for developers when it comes to figuring out how to use an API for complex operations. Maybe even examples of how the API works in certain real-world scenarios. Let’s assume you’ve got a financial application where the API handles the transaction process. You may write case studies breaking down the steps involved in the use case.
You may also include step-by-step tutorials that guide developers through complicated workflows. In such tutorials, you should include step-by-step commands, potential pitfalls to avoid, and troubleshooting tips for typical challenges developers might face as they proceed.
4. Use Visual Aids for Clarity
Drawing visual aids such as flow diagrams and UML models, helps designers present a tangle of operations in a more comprehensible form than a textual description. For example:
Flow diagrams: A flow diagram can present an end-to-end overview of an API workflow (eg, the process of validating a request from a user to access an API, from sending login credentials to receiving an access token).
Sequence diagrams: Suitable for illustrating the sequence of API calls and the flow of data between services or components.
UML diagrams: Useful for documenting complex data models or resource relationships in the system.
5. Address Performance and Scalability Considerations
When it comes to complicated operations, developers have to think about the performance considerations of the API. The main reason for this is that batch processing might suffer unless you tune it through best practices for wide datasets. Documentation should cover best practices for:
- Rate limits and throttling: Mention what the API’s rate limits are (so developers know how many requests they can send in a given period), and define throttling rules to prevent developers from sending too many requests at once.
- Optimizing API requests: Suggest methods for handling large data sets such as pagination or batching to reduce the number of API calls necessary in a specified workflow.
Streamline your API Documentation
Your API documentation is nonnegotiable because no matter how solid your API architecture is, if the people who work with your API can’t get any information about what it does, they’re not going to use it effectively.
Make sure to keep your documentation simple. Stick with thorough and complete descriptions. Know the right tools for the job, and always update your API. If you’re looking to improve your API documentation game, get in touch with us, and we’ll be more than happy to help you with a custom solution for your project.