Code documentation is the process that helps anyone writing software keep tabs on their code. It’s a hybrid of textual and pictorial clarifications describing or clarifying what a code base does and its usage. You may view it as annotations to your code.
Documenting your code makes it more readable, reproducible, and usable. Whether you have simple explanations of parts of your code, authoring and multi-page developer handbooks, or summarizing components of your application, you’re dealing with code documentation.
Other instances of documentation include API documentation, class, method, argument, and return value descriptions. Images, including sequence and entity-relationship diagrams and README files, are further examples of code documentation.
Why should you document your code? What are the benefits of doing so? Is there any reason to describe your choices and implementations after tasking coding sessions? This article explores what code documentation represents, what you should document, and how to do it right.
Importance of Documenting Code
You may be wondering why you should endure the hassle of documenting your code. But it’s not a needless exercise. Understanding the why makes it easier to appreciate the how of proper code documentation.
Anyone with any measure of experience in software development, engineering, or tech will agree that reading code soon becomes a more urgent task than writing it. After developing software for a while, you’ll find yourself reviewing previous versions of [even your own] code.
At other times, you’ll be poring over documentation from another source. It could be a team member’s docs or the code from third-party frameworks, libraries, and their examples.
These activities ultimately train us to become conscious of writing readable and maintainable code so that others will find it easier to understand our bits of ingenuity.
Here are two factors that should make documenting your code a priority.
1. Your team will become more valuable
The typical software development lifecycle features both technical and non-technical stakeholders. However, interaction and documentation are primarily through the technical documentation maintained for the project.
The number of parties involved in the project makes it imperative to ensure that the communication is understandable to both technical and non-technical people. The ability to target both groups with the same level of effectiveness within and outside your organization makes you an integral part of a software project.
Developing such an adaptable communication style in addition to your code improves your technical communication and employability.
2. Documentation makes your code more usable
Well-documented code has a high chance of reuse. Many times it determines how many people use a code library. It’s especially true with open-source, where more high-quality contributions are made to documented projects.
In fact, from the perspective of users, they’re more likely to prefer less powerful libraries bundled with superior documentation. That’s because the documentation of a codebase has more information than the code itself.
Documentation is a sign that a developer thinks it’s necessary to explain how their code works. Explaining their decisions in simple and accessible language helps more people to approach the codebase, learn it well, and expertly use it.
Users are happier if the project they’re exploring in their favorite programming language comes with thorough descriptions baked in.
Best Practices Your Team Should Adopt For Documenting Code
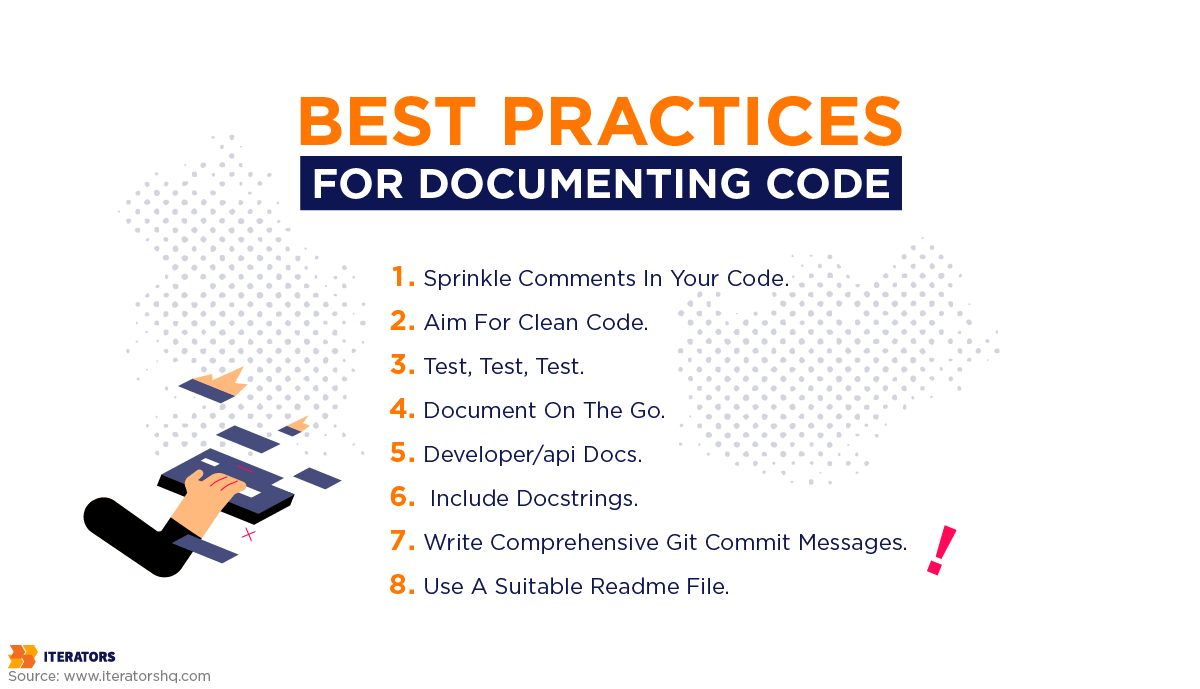
Now that you understand the reason for documenting your business logic right, you can also learn some industry-grade methods to keep your code readable and well-documented.
1. Sprinkle comments in your code
Comments don’t influence what happens when your code runs. They don’t belong in the scheme of events at runtime. However, they ensure that anyone going through your code can trace what’s happening.
If you don’t want to write comments in your code just for the sake of doing so, here are some helpful tips to keep in mind during the process:
- Be explanatory, and don’t merely restate what the code is doing.
- Be vivid with the why. Clearly describe what the code is doing and the specific step of the algorithm.
- Your comments should be accessible – they need to be as readable as possible. Tabs and other forms of whitespace are good tools for enhancing the clarity of your code.
- Improve your efficiency at writing comments by learning and using the tools and plugins present in your code editor or IDE. If you’re using VSCode, for instance, GhostDoc and AddJSDoc comments are examples of helpful documentation tools. The latter is popular among developers because it’s intuitive and easy to use.
The Mozilla Developer Network offers this helpful resource for more tips and tricks for writing effective comments.
2. Aim for clean code
Before writing any form of documentation, however, it’s necessary to work towards writing effective clean code.
If your code needs to be more explicit, it’s hard to write clean documentation from it. Properly format your code to ensure clarity of purpose and structure.
Where your code is hard to understand without comments, it’s a signal that you need to review your code and consider refactoring the complex bits to make them more manageable.
When developing production-level code, a logical and manageable directory or folder structure is non-negotiable. Use relevant naming standards for files, variables, and methods across the application.
Developers need to be conscious of following the DRY (Don’t Repeat Yourself) principle when writing code. In addition, you can keep your code garden tidy by maintaining standards in the project.
3. Test, test, test
Writing unit tests helps developers ensure that their code works as expected. It’s a way to sniff out bugs and prevent them from showing up in the future.
Every test case you write should let you know how your code is supposed to behave. Therefore, it instills confidence that no dreaded issues appear in the grand stage of production.
It’s preferable to use language- or framework-specific tools or libraries when writing test cases. Let’s say you’re working with Node.js or React – Jest is perfect for either. It enables easy mocking and code coverage since it’s fast and secure.
4. Document on the go
Good version control will help you track all document changes in your project. It’s best to write your comments in step with your code. You’ll be more accurate on the why of every decision and save valuable time trying to figure out what code you wrote a long time ago actually means.
5. Developer/API Docs
This type of documentation can be automatically generated from code comments. It’s widely used in organizations and is developed for software engineers to help them understand the details of the parts of the code they can use and how they should use them.
Here’s an example of this in the Ruby programming language:
import { add } from './lib/add';
// use the add function to add two numbers together
const answer: number = add(74, 3);
// answer will be 77
console.log(answer);
// Output: 77
6. Include docstrings in your code documentation
Docstrings help document functional code units such as functions or classes. By definition, they’re specialized multi-line descriptions occurring at the onset of a function definition.
With docstrings, you have a standard method for specifying the distinct parts of the function definition. Their form depends on the programming language used; in Python, they’re strings, whereas they’re comments in the R programming language.
Docstrings provide a link between your documentation and its components, keeping your documentation current. Someone using your library can access docstrings using the help(function name) format. They’re accessible even when you don’t open the source code files in another window.
The two available docstring types have unique guidelines for writing them. A functional docstring includes information on what role the function or class plays in the code, its parameters (and their types), and the outcomes of the code.
They may also contain information on common errors and the accompanying exceptions, descriptions of the function methodology, usage examples, and references to related functions and classes.
Structural docstrings, on the other hand, are clarifying comments packaged with standalone modules containing multiple functions. They’re concise and don’t repeat information found in a functional docstring.
Components of a structural docstring include a title, a short description, and essential usage elements not yet covered. They may also feature copyright information if third-party source code is part of the script besides academic citations, where necessary.
Your docstrings need to be current, too, as users might be using outdated information that no longer reflects the present realities of your program. Your docstrings should be available as soon as you write your code, in the same breath, or beforehand if you have determined your implementation. Edit your docstrings every time you alter the code and the functionality of your code.
Here are best practices on docstrings and related style guidelines.
7. Write comprehensive git commit messages
Version control is indispensable in software development. Your git commits messages to make your pull requests (or PRs) clear enough for the team to do any effective debugging.
In addition, they ensure that it’s easier to track implementations. Using a task or issue ID in your git commit messages is a way to identify a pushed feature and trace it afterward uniquely.
In learning how to write good git commit messages, it’s best to use the imperative present tense as follows: #294598 add shopping cart.
8. Use a suitable README file
A README file is a documentation of guidelines on how to deploy a software project. One common component of a README includes comprehensive instructions on installing and running a project.
These are the standard features of a README file:
- Project title
- Project description
- Licensing
- Versioning
- Instructions on installing and running the project in context
- The directory, or folder, structure and its peculiarities
- An outline of known issues
- Credits
The VSCode marketplace offers the Markdown Preview Enhanced to help you create a standard README file.
The Value of Self-Documenting Code
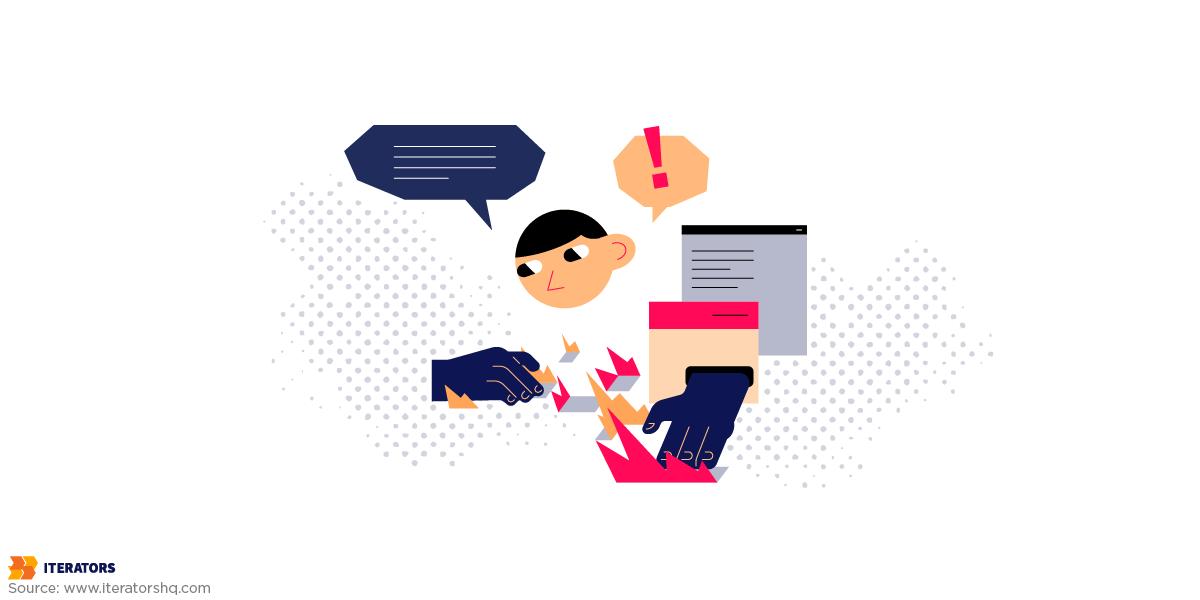
This point is deserving of its own section because if you’re going to be writing production-level code, then some things are a priority.
So, what’s there to know?
- Implement an intuitive and manageable directory or folder structure.
- Apply meaningful conventions in naming your files, variables, and other token elements in your project.
- Avoid writing redundant code. In other words, when you find yourself repeating significant chunks of your logic, you can generalize them by passing variable arguments.
- On occasion, your logic will be so convoluted that you’ll need help understanding what precisely you did only a few weeks or months later. Using comments here will help you to comprehend what’s going on quickly. However, it could be a better style to comment on every last line of code.
- Make your code more readable with appropriate formatting. Be consistent with the convention you use throughout the project, and tools such as prettier can be helpful.
- Perform regular code reviews as part of your development tasks. You’ll find room to improve and optimize your code during code review. You’ll also make opportunities to enhance performance and your documentation. It will spare you plenty of time down the road and get you ahead on the learning curve. Uncle Bob’s clean code series will help any developer.
Documenting Tech Stack and Environment Setup
Your tech stack combines various technologies to build and run applications or projects at your organization. Also known as solution stacks, they often comprise programming languages, frameworks, databases, frontend and backend tools, and applications linked through APIs.
Tech stacks need extensive documentation. A dedicated tech stack document or guide for an application helps everyone understand how to use the software, administer it, and integrate it with other systems.
The tech stack doc audience refers to anyone who needs to use the app, extend it, work with it, and discover how it fits into another tech stack ecosystem. Creating and maintaining a tech stack document is a collaborative effort where team members and the business system owner team, technical owner, and others participate in implementation and operations.
Each tech stack documentation lives inside the Business Owner’s Functional section of the documentation, alongside other Function-based handbook content.
On the other hand, a good product development team needs to codify in writing guidelines for setting up a development environment. New team members will find this resource valuable.
An environment setup documentation typically contains information on getting source code, running tests, and building the application. It also includes information on running the application on a new computer system.
While verbal communication helps deliver much of this information, no one has these details off-hand. Imagine memorizing customization settings, file paths, URLs, and so forth. Collecting them instead in one handy document makes setting up the development environment easier and faster.
It’s best to keep the setup requirements to a minimum by automating the dev environment and the specific build. Yet, it needs to be clear the means to get the source code and run that build while performing other necessary tasks. You may discover that you’re not actually automating everything.
The Value of Documenting Tech Stack and Environment Setup
There’s probably no need to document the tech stack and environment setup if everyone on the development team already knows how to build and release software. But, this thought process assumes that the team never changes.
In real life, team members are almost always in flux. In other words, old members go, and new ones come. One short-term project may keep the same team from start to finish. But, software is often extended – sometimes by functionality, other times by feature set. In such cases, the baton of maintenance or further development may change hands sooner than later.
On the flip side, imagine a long-term software project where a developer only stays on the team for a year. With time, there’ll be a team member rotation every few months.
Such alterations in the development team come with a disruption in development. You’ll have to deal with time lost on handover, developer differences, broken velocity calculations, and reduced productivity.
In addition, estimates will become less reliable and predictability reduced. These are things that documenting the tech stack, and environment setup can help to mitigate.
Benefits of documenting the tech stack and environment setup for new hires
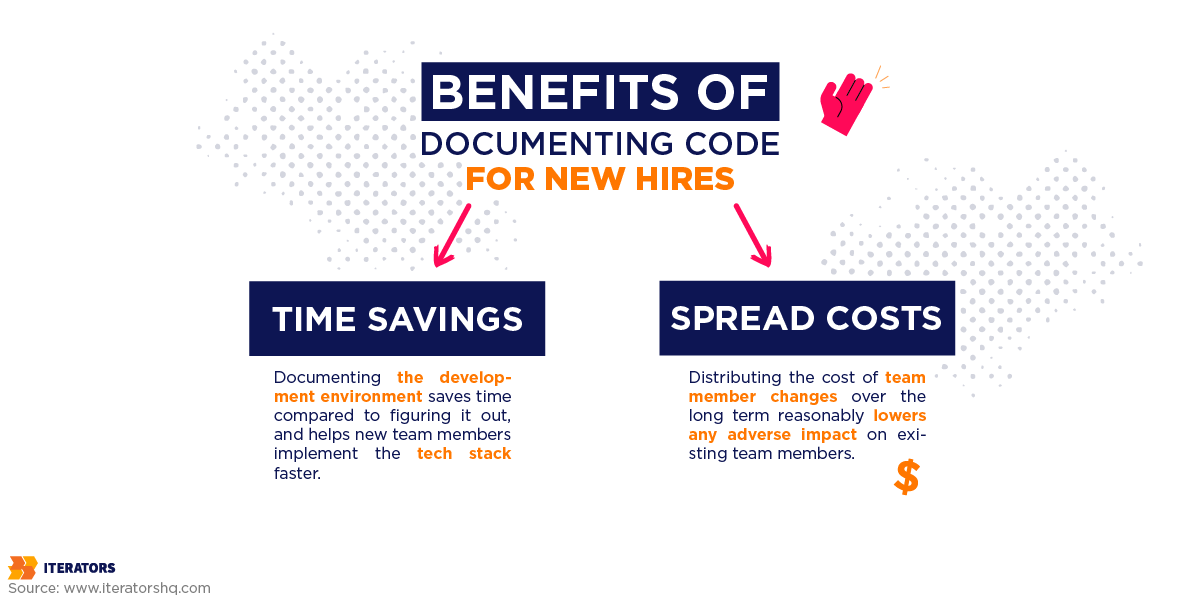
Software teams derive at least two benefits from written documentation as new team members navigate their first two weeks on the project. These include:
- Time Savings: It takes way less time to write development environment documentation than to figure it out. It takes even less time for new team members to implement the content of environment setup and tech stack docs than to figure out what’s going on in their new surroundings.
- Spread Costs: Distributing the cost of team member changes over the long term reasonably lowers any adverse impact on existing team members.
It’s nothing close to rocket science documenting a development environment. It’s less demanding than gathering product requirements. But, trying to create the development environment without the documentation could take significantly longer.
You want your team member to hit the ground running, and that’s precisely what documentation achieves. You won’t really need any team members to spend time helping them at the expense of higher-value work. Team members can use slack time on previous sprints to write documentation.
How to Document Tech Stack and Environment Setup
A README is the gold standard for capturing new team members’ knowledge. The process of creating valuable documentation for your tech stack and environment setup begins with an audit. Every effective tech stack and environment setup audit focuses on the business processes connected to each app instead of the app itself.
Get input from all stakeholders and app users. It includes directors, IT leads, team leaders, individual contributors who actively use the app, and new team members.
The facts you collect should focus on each software and the attendant business process while pinpointing users’ views on the effectiveness of each app for scheduled analysis. Subjects to discuss include:
- Business processes
- Apps teams use for daily tasks
- The business impact of each app and how each app affects employee experience and user experience
- After reviewing each app, your document needs to answer the following questions:
- How, where, and when does each software feature?
- How integral is the app in the business operation?
- What’s the cost of moving to a different system?
- Can an app meet more than one need, and what are those needs?
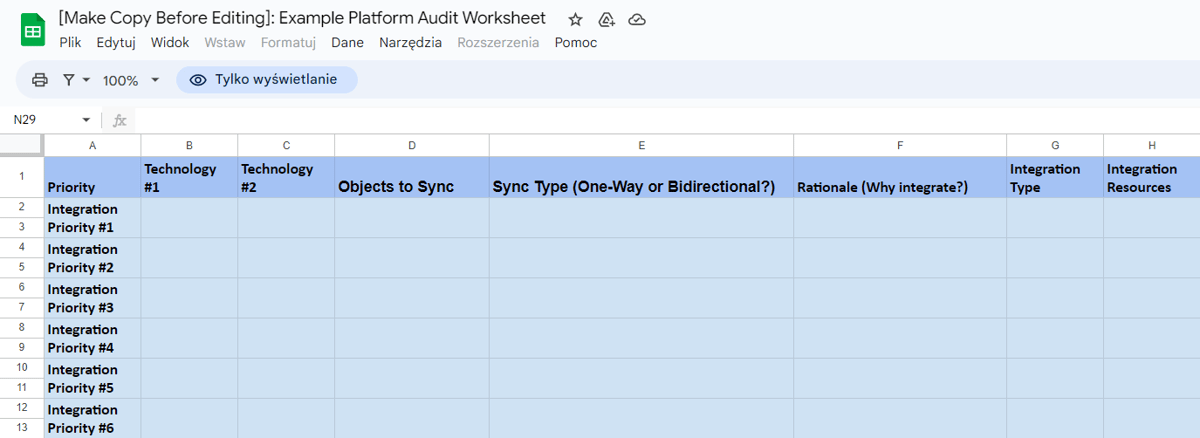
An audit worksheet such as this is helpful for recording your answers. Once you have your answers ready from all stakeholders, you can proceed to write the tech stack and environment setup documentation in line with general documentation best practices.
Tips For Effective Tech Stack and Environment Setup Documentation
The business analysis team is usually not responsible for overseeing the technology task for a software project. However, the team’s management tool comes in handy to organize the tech stack details across a range of products. It helps to reveal commonly used technology as well as duplicate functionality through various tools.
In a small- to medium-sized organization without centralized infrastructure management, you can introduce organization and complexity with as little overhead as possible.
The entire tech stack helps to manage licenses and external tools, enabling your organization in terms of economies of scale. Also, include tracking the sources of licensed components and their contract dates so that developers and infrastructure specialists don’t have to worry about this.
The tech stack docs for all products in an organization should follow the same outline. Some sections contain one or more entries per product containing product-specific information. Other sections are categorized by technology items such as database server and web server. Products using each item are associated with the specific item.
In the event that multiple products use different tech, say some use MariaDB and others Oracle, the tech stack includes entries for both MariaDB and Oracle. If products use different versions of either, the tech duly documents an entry for each version.
Common Pitfalls to Avoid In Documenting Code and Tech Stack
When you write documentation, it’s necessary to make sure that it’s effective. It turns out this is one area where junior developers should really spend a lot of time. Thankfully, they can learn from folks with a bit more experience. That’s one way to avoid making rookie doc mistakes for too long.
What are these common pitfalls anyway?
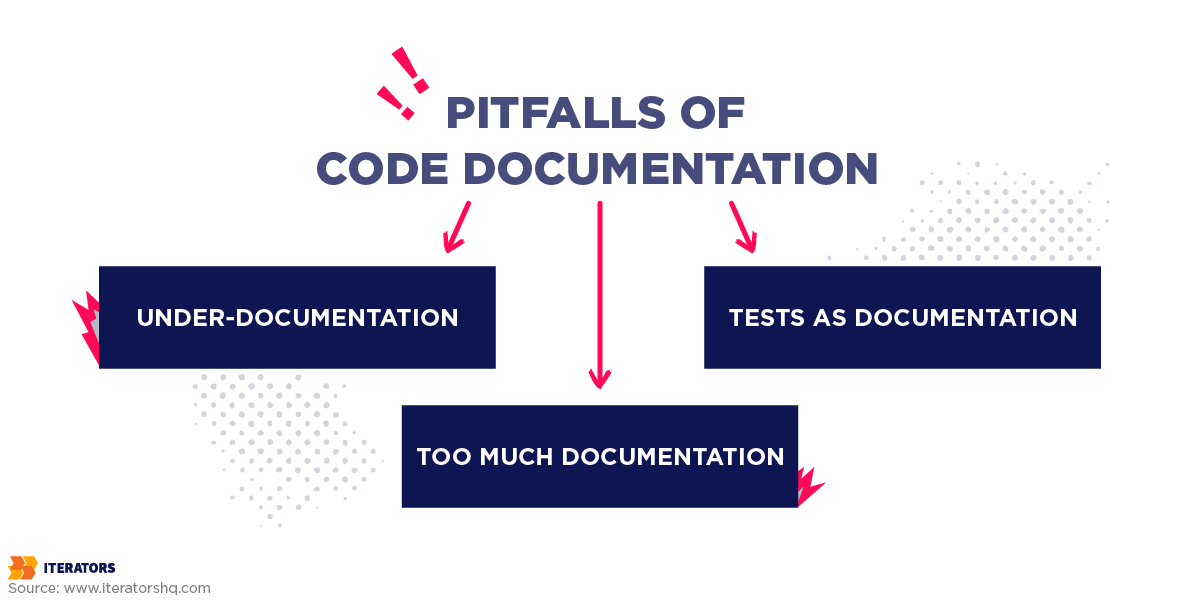
Under-documentation
Many attempts at code documentation only manage to rehash obvious or already visible information. The goal of the documentation exercise isn’t merely to add to a function a block that repeats the name of the function, its parameter names, and their types. It clearly doesn’t do anything worthy of note unless it advances your ability to build a better software product.
Developers can read the signature of a method (its name and parameters) in a few seconds. That’s not what documentation is all about.
Your documentation reflects what the function does and its role within the application (the why). In other words, it should outline how each parameter changes the behavior of the function and by implication, the user experience.
Too much documentation
Just as it’s possible to document less than is necessary, you may also fall into the trap of over-documentation. In order to beef up documentation, some tend to write an explanation for every last token or bit of logic in their code.
But do you really need to detail all the minutiae that went into creating your function?
There’s no point in spending as much time writing documentation as you’ve taken to develop the function. Such documentation may be hard to understand and may take as much time as it takes to read the code. Remember that time is in short supply.
Good technical documentation basically addresses the what and the why. The especially tricky parts of your code are the ones you should focus on in your documentation – it’s easy to see their value.
Your customers only care about the what and why if the final product is worth paying for.
Using tests alone as a means of documentation
Many devs believe that unit tests should be the preferred form of documentation. Tests are great, but this view is flawed primarily because, just like code, unit tests can be hard to read.
A developer should not have to go through the painful exercise of locating your unit tests and reading them all in a bid to understand your code. In many cases, they need more patience to do so.
Therefore, tests are good and provide a sound support system in any codebase, but they aren’t a substitute for good documentation.
Case Studies of Successful Code and Tech Stack Documentation
Successful code documentation is deserving of skill and effort. Having cited the near-ubiquitous example of the README file, we need now look at further examples of technical documentation that work.
Here are a few excellent examples of code documentation that works.
1. GoCardless
The GoCardless service handles recurring payments with Direct Debits. Its technical documentation is inviting as it helps users get started and comes with many code samples to help you get your feet wet.
The sheer number of examples in the documentation means developers will hardly request assistance from GoCardless support.
2. Ruby on Rails
With a solid reputation as one of the foremost web development frameworks, Ruby on Rails partly owes its fame to its robust inline documentation.
If you’re wondering whether or not to use a method, you can quickly scour the source code to see the method’s comprehensive documentation. This documentation system helps people to learn Rails fast.
3. Stripe
Stripe could rightly claim to be “the king of small business payments.” The company prides itself on being a developer-first platform and supports its claim with examples and use cases.
Stripe’s massive documentation is where your search engine is likely to take you for queries related to payments development, helping them win a lot of business in the process. You can replicate this formula to grow your business.
4. TextExpander
The README for TextExpander Touch SDK is a classic example of successful code documentation. It’s easy to access and use when you need to add TextExpander functionality to your iOS application.
The Role of Code Documentation In Project Management and Maintenance
Contrary to product-based technical documentation, the value of project-based technical documentation is of internal rather than external impact. This type of documentation explains to your team the process of making your software product.
Technical documentation supports project management by clarifying the scope of the project. It also outlines in detail the steps and processes your team should replicate where necessary.
Project-based technical documentation includes:
- Project timelines;
- Internal meeting notes and reports; and
- Product requirements
In essence, code documentation provides the guardrails for effective project management and maintenance.
Conclusion
Writing excellent code documentation benefits the developer (or development team), the project, other stakeholders, and users. Since it’s a loop that caters to disparate interests, improving your technical writing and documentation skills is essential.
When you write documentation right and promptly, you oil the wheels of developer collaboration. Just as it applies to your code, Don’t Repeat Yourself is golden advice when writing the docs.
Besides using well-formatted docstrings and pushing updates to your README file, you’ll do well to follow the best practices shared in this article. See you on the other side of the docs.
Interspersing good documentation with your code will help you to clarify your thinking in the present and positively impact your team over the long term. If your documentation helps stakeholders, it’s easier to win their long-term buy-in for your project.